Create a teamplate app.component.html
Configure the HttpClientModule src/app/app.module.ts
Categories: Angular
<div class="container">
<div class="row">
<div class="col-md-6 offset-md-3">
<h3>Choose File</h3>
<form (ngSubmit)="onSubmit()">
<div class="form-group">
<input type="file" name="image" />
</div>
<div class="form-group">
<button class="btn btn-primary">Submit</button>
</div>
</form>
</div>
</div>
</div>
Configure the HttpClientModule src/app/app.module.ts
import { HttpClientModule } from '@angular/common/http';Add HttpClientModule to imports array under @NgModule
imports: [Now open the app.component.ts file and put the below code on it
HttpClientModule
]
fileData: File = null;After the changes our app.component.ts file will looks like this
constructor(private http: HttpClient) { }
fileProgress(fileInput: any) {
this.fileData = <File>fileInput.target.files[0];
}
onSubmit() {
const formData = new FormData();
formData.append('file', this.fileData);
this.http.post('url/to/your/api', formData)
.subscribe(res => {
console.log(res);
alert('SUCCESS !!');
})
}
import { Component } from '@angular/core';First of all open the app.component.ts file and put the below import
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'helloworld';
fileData = null;
constructor(private http: HttpClient) { }
ngOnInit() {
}
fileProgress(fileInput: any) {
this.fileData = <File>fileInput.target.files[0];
}
onSubmit() {
const formData = new FormData();
formData.append('file', this.fileData);
this.http.post('url/to/your/api', formData)
.subscribe(res => {
console.log(res);
alert('SUCCESS !!');
})
}
}
import { HttpClient, HttpEventType } from '@angular/common/http';Then you just need to add reportProgress to true and set the observe to events in the config like we have below. You will get all the events on subscribe
this.http.post('url/to/your/api', formData, {
reportProgress: true,
observe: 'events'
})
.subscribe(events => {
if(events.type == HttpEventType.UploadProgress) {
console.log('Upload progress: ', Math.round(events.loaded / events.total * 100) + '%');
} else if(events.type === HttpEventType.Response) {
console.log(events);
}
})
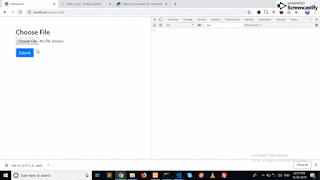
Categories: Angular
What type is formData when passed to a web api? I have a sql server table whereby the image to be store is defined as [ImageData] [varbinary](max) NOT NULL. What is the compatible property type that I should have in the web api so that the insert is successful?
ReplyDelete