You can create a nested routing by defining child routes using the children property of a route (alongside a path and component properties). You also need to add a nested router-outlet in the HTML template related to the component linked to the parent route (In our case it's the admin route).
To create nested routing, you need to create a routing submodule for the module you want to provide routing, you next need to define a parent route and its child routes and provide them to the router configuration via a forChild() method.
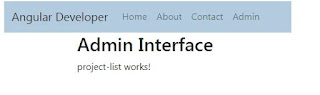
Creating an Angular 9 Routing Module
Let's see this step by step. First, inside the admin module, create an admin-routing.module.ts file and add a submodule for implementing child routing in our admin module:
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { ProjectComponent } from './project/project.component';
import { ProjectListComponent } from './project-list/project-list.component';
import { ProjectCreateComponent } from './project-create/project-create.component';
import { ProjectUpdateComponent } from './project-update/project-update.component';
const routes: Routes = [
{
path: 'admin',
component: ProjectComponent,
children: [
{
path: 'list',
component: ProjectListComponent
},
{
path: 'create',
component: ProjectCreateComponent
},
{
path: 'update',
component: ProjectUpdateComponent
}
]
}
];
@NgModule({
imports: [RouterModule.forChild(routes)],
exports: [RouterModule]
})
export class AdminRoutingModule { }
This is an example of a module which has imports and exports meta information;
The imports array which contains the modules that we need to import and use in the current module. In this case it's RouterModule.forChild(routes),
The exports array which contains what we need to export.
Adding Child Routes to the Angular 9 Router Module
In order to provide our child routes to the router module, we use the forChild() method of the module because we want to add routing in the admin submodule. if this is used in root module you need to use the forRoot() method instead. See more differences of forChild() vs forRoot() from the official docs.
The forChild() and forRoot() methods are static methods that are used to configure modules in Angular. They are not specific to RouterModule.
We are creating a parent admin route and its own child routes using the children property of the route which takes an array of routes.
You can respectively access the ProjectListComponent, ProjectCreateComponent and ProjectCreateComponent using the /admin/list, /admin/create and /admin/update paths.
Next, open the src/app/admin/admin.module ts file and import the routing module:
// [..]
import { AdminRoutingModule } from './admin-routing.module';
@NgModule({
// [...]
imports: [
CommonModule,
AdminRoutingModule
]
})
export class AdminModule { }
Next open the src/app/admin/project/project.component html file and add a nested router outlet:
<h2>Admin Interface</h2>
<router-outlet></router-outlet>
This is a nested router-outlet that will be only used to render the components of the admin module i.e ProjectListComponent, ProjectCreateComponent and ProjectCreateComponent.
Note: If you don't add a nested router outlet in the parent route, child components will be rendered in the parent router outlet of the application.
Next in the src/app/header/header.component.html file, add a link to access the admin interface:
<li class="nav-item">
<a class="nav-link" routerLink="/admin/list">Admin</a>
</li>
At this point, if you click on the admin link in the header, you should see the following interface:
No comments:
Post a Comment