Kotlin files have a .kt suffix.
Package declaration
The beginning of the code file is generally the package declaration:
In the above example, the full name of test () is com.tienanhvn.main.test, and the full name of Tienanhvn is com.tienanhvn.main.Tienanhvn.
If no package is specified, the default package is the default .
Default import
There are multiple packages that will be imported into each Kotlin file by default:
Function definition
The function definition uses the keyword fun, and the parameter format is: Parameter: Type
Variable-length parameters of functions can be identified with the vararg keyword:
Examples of lambda expression usage:
// Test
Variable variable definition: var keyword
The compiler supports automatic type determination, which means that the type can be specified without declaration and is determined by the compiler.
Kotlin supports single-line and multi-line comments. Examples are as follows:
// This is a single line comment
/ * This is a multi-line
Block comment. * /
Unlike Java, block comments in Kotlin allow nesting.
String template
Kotlin's empty security design requires null judgment for the parameters that can be declared null. There are two processing methods. Add after the field !! Like Java, throw an empty exception, add another field after? Can not do Processing return value is null or cooperate ?: short judgment processing
// The type followed by? Indicates that it can be null var age : String ? = "23" // Throw a null pointer exception
When the string content in str is not an integer, return null:
fun parseInt ( str : String ): Int ? { // ... }
The following example shows how to use a function that returns null:
We can use the is operator to detect whether an expression is an instance of a certain type (similar to the instanceof keyword in Java).
Interval expression having the form of the operator .. The rangeTo in function and combined! In form.
Intervals are defined for any comparable type, but for integer primitive types, it has an optimized implementation. Here are some examples of using intervals:
Package declaration
The beginning of the code file is generally the package declaration:
package com . tienanhvn . mainKotlin source files do not need matching directories and packages. Source files can be placed in any file directory.
import java . util . *
fun test () {} class Tienanhvn {}
In the above example, the full name of test () is com.tienanhvn.main.test, and the full name of Tienanhvn is com.tienanhvn.main.Tienanhvn.
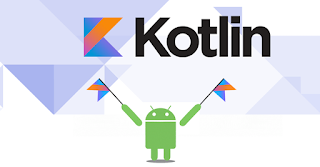
If no package is specified, the default package is the default .
Default import
There are multiple packages that will be imported into each Kotlin file by default:
- kotlin. *
- kotlin.annotation. *
- kotlin.collections. *
- kotlin.comparisons. *
- kotlin.io. *
- kotlin.ranges. *
- kotlin.sequences. *
- kotlin.text. *
Function definition
The function definition uses the keyword fun, and the parameter format is: Parameter: Type
fun sum ( a : Int , b : Int ): Int { // Int parameter, return value Int return a + bThe expression as the function body, the return type is automatically inferred:
}
fun sum ( a : Int , b : Int ) = a + bFunctions with no return value (similar to void in Java):
public fun sum ( a : Int , b : Int ): Int = a + b // public methods must explicitly write the return type
fun printSum ( a : Int , b : Int ): Unit { print ( a + b ) }Variable length parameter function
// If it returns Unit type, it can be omitted (the same is true for public methods): public fun printSum ( a : Int , b : Int ) { print ( a + b ) }
Variable-length parameters of functions can be identified with the vararg keyword:
fun vars ( vararg v : Int ) { for ( vt in v ) { print ( vt ) } }lambda (anonymous function)
// Test
fun main ( args : Array < String >) {
vars ( 1 , 2 , 3 , 4 , 5 ) // output 12345 }
Examples of lambda expression usage:
// Test
fun main ( args : Array < String >) {Define constants and variables
val sumLambda : ( Int , Int ) -> Int = ( x , y- > x + y )
println ( sumLambda ( 1 , 2 )) // output 3 }
Variable variable definition: var keyword
var <identifier> : <type> = <initialization value>Definition of immutable variables: val keyword, variables that can only be assigned once (similar to final modified variables in Java)
val <identifier> : <type> = <initialization value>Both constants and variables can have no initialization value, but they must be initialized before reference
The compiler supports automatic type determination, which means that the type can be specified without declaration and is determined by the compiler.
val a : Int = 1Comment
val b = 1 // The system automatically infers the variable type as Int
val c : Int // If it is not initialized at the time of declaration, you must provide the variable type
c = 1 // Explicit assignment
var x = 5 // The system automatically infers that the variable type is Int
x + = 1 // The variable can be modified
Kotlin supports single-line and multi-line comments. Examples are as follows:
// This is a single line comment
/ * This is a multi-line
Block comment. * /
Unlike Java, block comments in Kotlin allow nesting.
String template
$ Represents a variable name or variable valueNULL check mechanism
$ varName represents the variable value
$ {varName.fun ()} means the return value of the variable method:
var a = 1 // Simple name in the template:
val s1 = "a is $ a"
a = 2 // any expression in the template:
val s2 = "$ {s1.replace (" is "," was ")}, but now is $ a"
Kotlin's empty security design requires null judgment for the parameters that can be declared null. There are two processing methods. Add after the field !! Like Java, throw an empty exception, add another field after? Can not do Processing return value is null or cooperate ?: short judgment processing
// The type followed by? Indicates that it can be null var age : String ? = "23" // Throw a null pointer exception
val ages = age !!. ToInt () // Return nullWhen a reference may be a null value, the corresponding type declaration must be explicitly marked as nullable.
val ages1 = age ?. ToInt without processing () // age is empty and return -1
val ages2 = age ?. toInt () ?: - 1
When the string content in str is not an integer, return null:
fun parseInt ( str : String ): Int ? { // ... }
The following example shows how to use a function that returns null:
fun main ( args : Array < String >) { if ( args . size < 2 ) { print ( "Two integers expected" ) return }Type detection and automatic type conversion
val x = parseInt ( args [ 0 ])
val y = parseInt ( args [ 1 ]) // Using `x * y` directly will cause an error because they may be null. If ( x ! = Null && y ! = Null ) { // After performing a null value check, the types of x and y are automatically converted to non-null variables print ( x * y ) } }
We can use the is operator to detect whether an expression is an instance of a certain type (similar to the instanceof keyword in Java).
fun getStringLength ( obj : Any ): Int ? { if ( obj is String ) { // After doing type judgment, obj will be automatically converted to String type return obj . lengthor
} by the system
// There is another method here, which is different from instanceof in Java, use! Is // if (obj! Is String) { // // XXX //}
// obj here is still Any type reference return null }
fun getStringLength ( obj : Any ): Int ? { if ( obj ! is String ) return null // In this branch, the type of `obj` is automatically converted to` String` return obj . lengthEven ok
}
fun getStringLength ( obj : Any ): Int ? { // On the right side of the `&&` operator, the type of `obj` is automatically converted to` String` if ( obj is String && obj . length > 0 ) return obj . lengthInterval
return null }
Interval expression having the form of the operator .. The rangeTo in function and combined! In form.
Intervals are defined for any comparable type, but for integer primitive types, it has an optimized implementation. Here are some examples of using intervals:
for ( i in 1 .. 4 ) print ( i ) // output "1234"Example test
for ( i in 4 .. 1 ) print ( i ) // output nothing
if ( i in 1 .. 10 ) { // equivalent to 1 <= i && i <= 10
println ( i ) }
// Use step to specify the step size for ( i in 1 .. 4 step 2 ) print ( i ) // output "13"
for ( i in 4 downTo 1 step 2 ) print ( i ) // output "42"
// Use the until function to exclude the end element for ( i in 1 until 10 ) { // i in [1, 10) excludes 10
println ( i ) }
fun main ( args : Array < String >) { print ( "Cycle output:" ) for ( i in 1 .. 4 ) print ( i ) // print "1234"Output result:
println ( "\ n ------- --------- " ) print ( " Set step size: " ) for ( i in 1 .. 4 step 2 ) print ( i ) // prints" 13 "
println ( "\ n ----------------" ) print ( "use downTo:" ) for ( i in 4 downTo 1 step 2 ) print ( i ) // prints "42"
println ( "\ n ----------------" ) print ( "Use until:" ) // Use the until function to exclude the end element for ( i in 1 until 4 ) { // i in [1, 4) excludes 4 print ( i ) } ) }
Loop output: 1234 ---------------- Set step size: 13 ---------------- Use downTo : 42 ----- ----------- Using until : 123 ----------------
No comments:
Post a Comment