In the above program to Check Whether a Number is Even or Odd, a Scanner object, reader is created to read a number from the user's keyboard. The entered number is then stored in a variable num.
Now, to check whether num is even or odd, we calculate its remainder using % operator and check if it is divisible by 2 or not.
For this, we use if...else statement in Java. If num is divisible by 2, we print num is even. Else, we print num is odd.
We can also check if num is even or odd by using if...else as an expression.
Example 1:
Now, to check whether num is even or odd, we calculate its remainder using % operator and check if it is divisible by 2 or not.
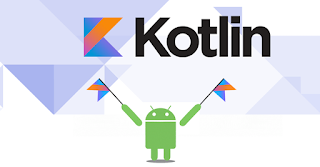
For this, we use if...else statement in Java. If num is divisible by 2, we print num is even. Else, we print num is odd.
We can also check if num is even or odd by using if...else as an expression.
Example 1:
import java.util.*When you run the program, the output will be:
fun main(args: Array<String>) {
val reader = Scanner(System.`in`)
print("Enter a number: ")
val num = reader.nextInt()
if (num % 2 == 0)
println("$num is even")
else
println("$num is odd")
}
Enter a number: 12Example 2:
12 is even
import java.util.*When you run the program, the output will be:
fun main(args: Array<String>) {
val reader = Scanner(System.`in`)
print("Enter a number: ")
val num = reader.nextInt()
val evenOdd = if (num % 2 == 0) "even" else "odd"
println("$num is $evenOdd")
}
Enter a number: 13
13 is odd
No comments:
Post a Comment