In Android, AutoCompleteTextView is an editable text view, used to display a list of suggestions based on user input. The list of suggestions will be displayed as a drop-down menu from which users can select an item to replace the content of the text box.
AutoCompleteTextView is a subclass of EditText class, so we can inherit all the properties of EditText in AutoCompleteTextView based on our requirements.The following is a representative image of using AutoCompleteTextView in Android applications.
Create AutoCompleteTextView in File Layout
The following is a sample way to define an AutoCompleteTextView in XML layout file in an Android application.
<?xml version="1.0" encoding="utf-8"?>Create the AutoCompleteTextView control in the active file
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<AutoCompleteTextView
android:id="@+id/autoComplete_Country"
android:layout_width="fill_parent"
android:layout_height="wrap_content" />
</LinearLayout>
In Android example, we can programmatically create the AutoCompleteTextView control in the activity file to display a list of suggestions based on the text the user has entered.
The following is an example of creating a flexible how to use AutoCompleteTextView control in the active file.
LinearLayout l_layout = (LinearLayout) findViewById(R.id.linear_Layout);Set Text of Android AutoCompleteTextView
AutoCompleteTextView actv = new AutoCompleteTextView(this);
l_layout.addView(actv);
In Android, we can place the text of the AutoCompleteTextView example control using the CHAPTERAd set () method in the Activity file.
The following is an example of AutoCompleteTextView associated data in the activity file using the CHAPTERAd set () method.
String[] Countries = { "India", "USA", "Australia", "UK", "Italy", "Ireland", "Africa" };
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_dropdown_item_1line, Countries);
AutoCompleteTextView actv = AutoCompleteTextView)findViewById(R.id.autoComplete_Country);
actv.setAdapter(adapter);
How to AutoCompleteTextView example
Following is the example of defining AutoCompleteTextView control in LinearLayout to bind the data to defined control using data adapter and getting the selected list item value in android application.
Create a new android application using android studio and give names as AutoCompleteTextViewExample. In case if you are not aware of creating an app in android studio check this article Android Hello World App.
Now open an activity_main.xml file from \res\layout path and write the code like as shown below
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="20dp"
android:paddingRight="20dp"
android:orientation="vertical"
android:id="@+id/linear_Layout">
<AutoCompleteTextView
android:id="@+id/ac_Country"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="100dp"
android:hint="Enter Country Name"/>
</LinearLayout>
Creata a class MainActivity.java
public class MainActivity extends AppCompatActivity {
String[] Countries = { "India", "USA", "Australia", "UK", "Italy", "Ireland", "Africa" };
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this,
android.R.layout.simple_dropdown_item_1line, Countries);
AutoCompleteTextView actv = (AutoCompleteTextView)findViewById(R.id.ac_Country);
actv.setThreshold(1);
actv.setAdapter(adapter);
actv.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Toast.makeText(getApplicationContext(), "Selected Item: " + parent.getSelectedItem(), Toast.LENGTH_SHORT).show();
}
});
}
}
Output of Android AutoCompleteTextView Example
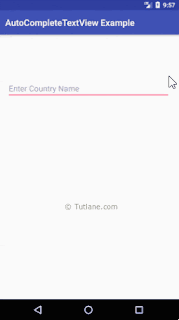
No comments:
Post a Comment