Install webpack loaders
Run the following command to install the npm packages required to convert LESS styles into CSS, then load the CSS styles and inject them into the DOM.
Create a LESS stylesheet named app.less alongside your root Angular app component (/src/app/app.component.ts) with some simple styles for testing, for example:
Update your webpack.config with the following module rules so webpack knows how to process *.less files.
Import global stylesheet into Angular app component
Import the app.less global stylesheet into your root Angular app component (/src/app/app.component.ts) with the following line.
Run the following command to install the npm packages required to convert LESS styles into CSS, then load the CSS styles and inject them into the DOM.
npm i --save-dev css-loader less less-loader style-loaderCreate a global LESS / CSS stylesheet
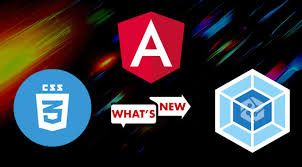
Create a LESS stylesheet named app.less alongside your root Angular app component (/src/app/app.component.ts) with some simple styles for testing, for example:
body {Add module rules to webpack config
background-color: #1abc9c;
}
Update your webpack.config with the following module rules so webpack knows how to process *.less files.
{This is the complete webpack config file from the above example app after the style loaders have been added:
test: /\.less$/,
use: [
{ loader: 'style-loader' },
{ loader: 'css-loader' },
{ loader: 'less-loader' }
]
}
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = {
entry: './src/main.ts',
resolve: {
extensions: ['.ts', '.js']
},
module: {
rules: [
{
test: /\.ts$/,
use: ['ts-loader', 'angular2-template-loader']
},
{
test: /\.(html|css)$/,
use: 'raw-loader'
},
{
test: /\.less$/,
use: [
{ loader: 'style-loader' },
{ loader: 'css-loader' },
{ loader: 'less-loader' }
]
}
]
},
plugins: [
new HtmlWebpackPlugin({ template: './src/index.html' })
],
devServer: {
historyApiFallback: true
}
}
Import global stylesheet into Angular app component
Import the app.less global stylesheet into your root Angular app component (/src/app/app.component.ts) with the following line.
import './app.less';This is the complete app.component.ts file from the above example app after the global stylesheet has been imported:
import { Component } from '@angular/core';source : https://jasonwatmore.com/post/2019/09/04/angular-webpack-how-to-add-global-css-styles-to-angular-with-webpack
import './app.less';
@Component({ selector: 'app', templateUrl: 'app.component.html' })
export class AppComponent {}
No comments:
Post a Comment